11. Pruebas y debugging¶
Para generar las pruebas usaremos pytest
11.1. Instalación¶
En Pycharm vamos a Pycharm -> Preferences -> Proyect: code -> Project Interpreter -> +
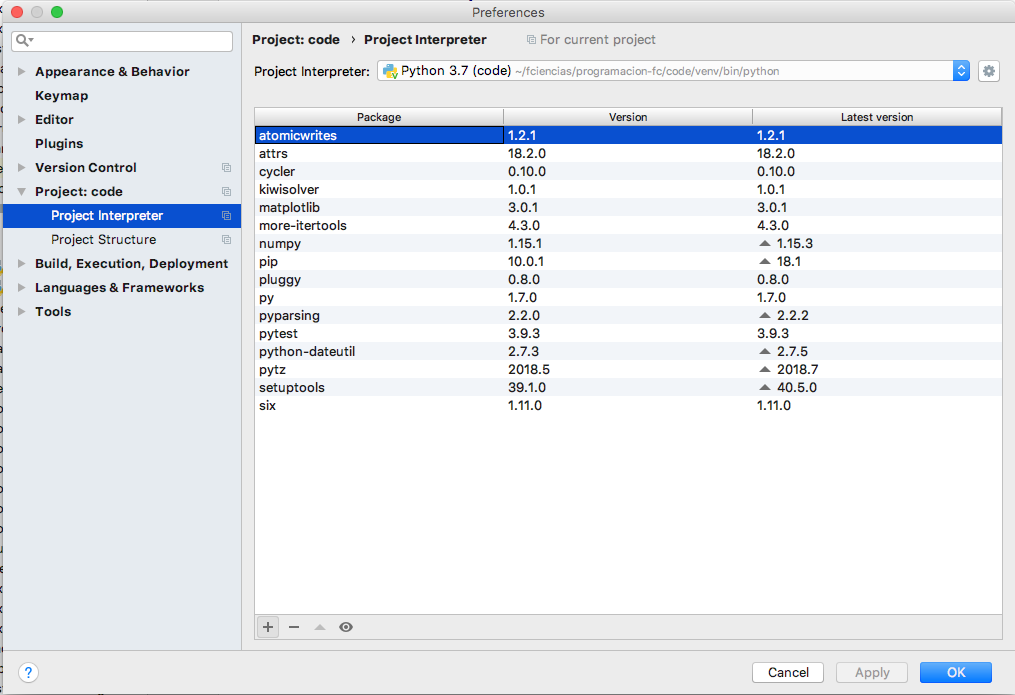
Escribimos pytest, lo seleccionamos y por ultimo damos clic en el botón “Install package”
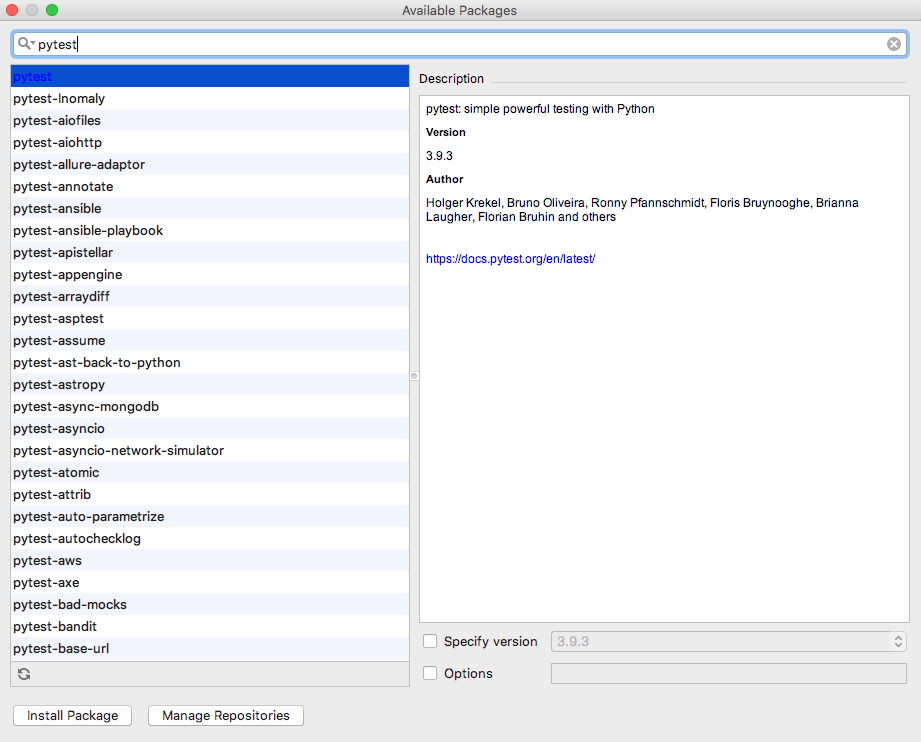
Debemos indicarle a Pycharm que usaremos pytest Pycharm -> Preferences -> Tools -> Python Integrated Tools
En la sección de testing seleccionamos pytest
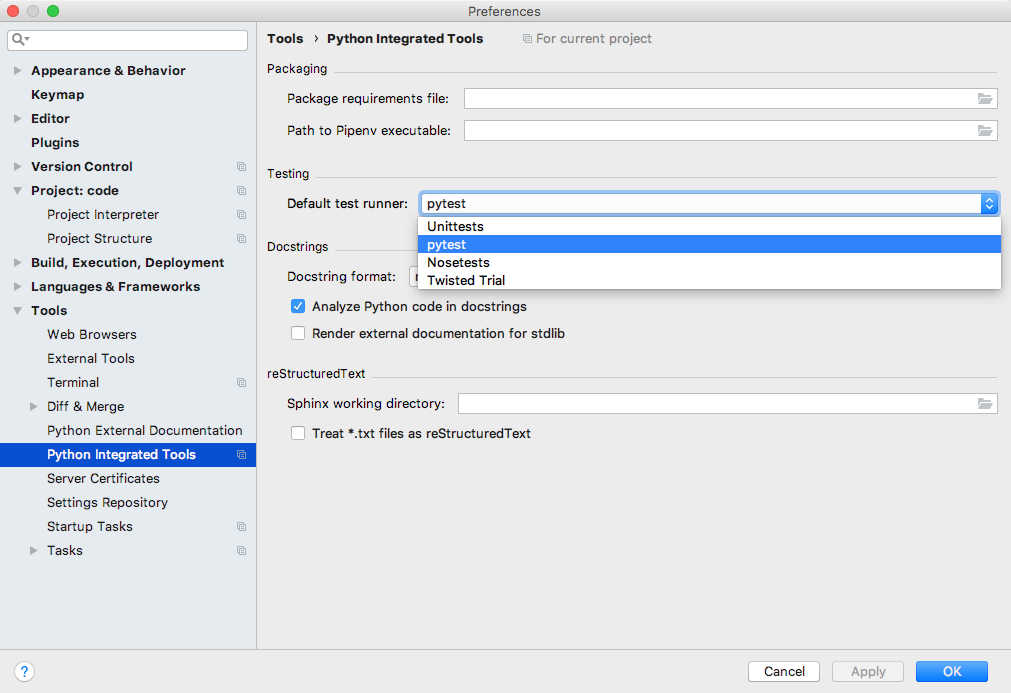
pruebas/tests/test_votacion.py¶
from pruebas.votacion import Votacion
def test_votar():
v = Votacion()
v.votar('A', 'X')
assert v.total() == 1
v.votar('A', 'X')
assert v.total() == 1
v.votar('A', 'Y')
assert v.total() == 1
v.votar('AA', 'Y')
assert v.total() == 2
def test_total():
v = Votacion()
assert v.total() == 0
v.votar('A', 'X')
assert v.total() == 1
v.votar('B', 'X')
assert v.total() == 2
v.votar('A', 'Y')
assert v.total() == 2
def test_votos():
v = Votacion()
for i in range(15):
v.votar('A' * i, 'X')
if i % 5 == 0:
v.votar('B' * i, 'Y')
assert v.votos('X') == 15
assert v.votos('Y') == 2
def test_ganador():
v = Votacion()
for i in range(15):
v.votar('A' * i, 'X')
if i % 5 == 0:
v.votar('B' * i, 'Y')
assert v.ganador() == 'X'
pruebas/votacion.py¶
from datetime import datetime
from datetime import timedelta
class Votacion:
def __init__(self):
self.urna = {'X': [], 'Y': []}
def votar(self, curp, opcion):
if opcion in self.urna:
yavoto = False
for k in self.urna:
if curp in self.urna[k]:
yavoto = True
if not yavoto:
self.urna[opcion].append(curp)
def total(self):
suma = 0
for key in self.urna:
valor = self.urna[key]
suma += len(valor)
return suma
def votos(self, opcion):
if opcion in self.urna:
return len(self.urna[opcion])
return 0
def ganador(self):
maximo = ''
for k in self.urna:
if self.votos(k) > self.votos(maximo):
maximo = k
return maximo
if __name__ == '__main__':
vot = Votacion()
fechalimite = datetime.now() + timedelta(minutes=1)
while datetime.now() < fechalimite:
curp = input('Cual es tu curp: ')
opcion = input('Caul es tu opcion: "X" o "Y"? ')
vot.votar(curp, opcion)
ganador = vot.ganador()
print('El gandor es %s con %s votos' % (ganador, vot.votos(ganador)))
Para ejecutar las pruebas ejecutamos: Run “pytest”.

Ahora podemos garantizar que el programa sigue funcionando como se espera si hacemos algún cambio en el código, por ejemplo:
podemos reescribir la función total()
de la siguiente manera:
def total(self):
return sum([len(v) for v in self.urna.values()])
la función votos()
:
def votos(self, opcion):
return len(self.urna.get(opcion, []))
la función ganador()
:
def ganador(self):
maximo = max([(len(v), k) for k,v in self.urna.items()])
return maximo[1]
la función votar()
:
def votar(self, curp, opcion):
repetido = [k for k, v in self.urna.items() if curp in v]
if not repetido and opcion in self.urna:
self.urna[opcion].append(curp)